Scripting Notes: The Accounts Class
In the Notes scripting dictionary, the account class is found in the Notes suite. It contains a few standard properties: name and id, and contains only one element, folders.
account n : A Notes account.
elements
contains: folders; contained by application.
properties
name (text, r/o) : The name of the account.
id (text, r/o) : The unique identifier of the account.
responds to
count, exists
Local or Online Accounts
By default, Notes provides access to either online accounts or local accounts. If the current user does not have an active online account (such as iCloud) in the Mail, Contacts & Calendars system preference pane, or Notes is not a selected service in the active account, then Notes will display only the local On My Mac account, and all notes will be stored locally on the computer. However, if iCloud or another online account is active, then Notes will display all the online accounts that have their Notes service checked, and will no longer display the On My Mac account.
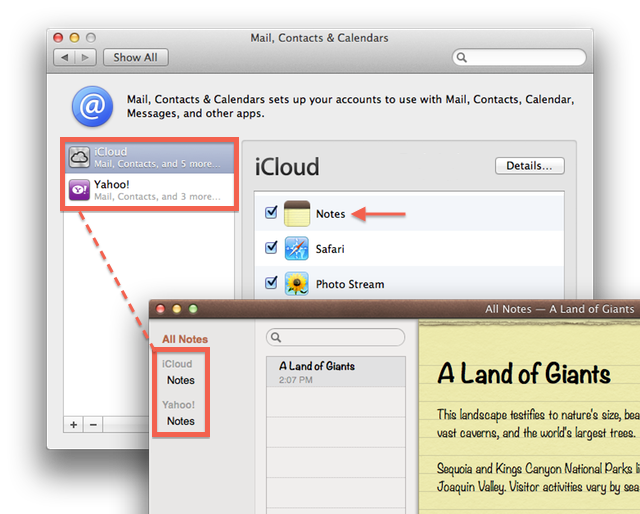
Notes will display every online account whose Notes service is active.
The value of the account name property can be used to determine if there are active online accounts:
In order for scripts to accommodate with the option of multiple active accounts, place the following sub-routine in your scripts. If there is only one active account, the sub-routine automatically returns the name of the current account. But if there exists more than one active account, the sub-routine will present a dialog to the user from which they can choose the account to target with the script.

This example demonstrates how to get the name of the targeted account.
Targeting Notes in the Account Class
Note that the account class does not contain the notes class as an element. This means that global queries or actions targeting notes cannot be done by addressing the account object.
For example, a script asking an account for the number of notes it contains will fail:
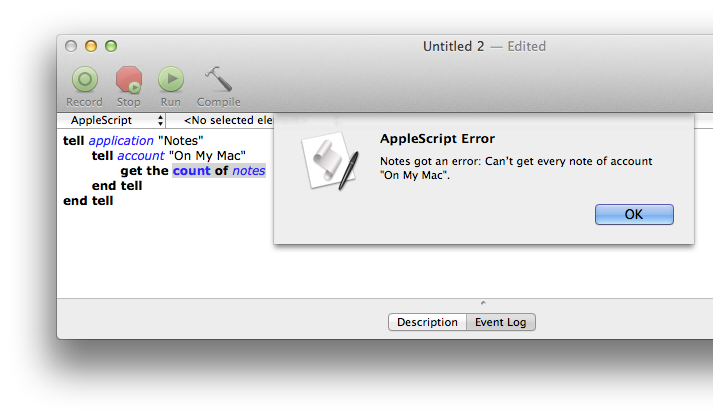
However, the same script will work if instead of requesting the number of notes in the account, the request asks for the count of notes in all of its folders (which are elements of an account):
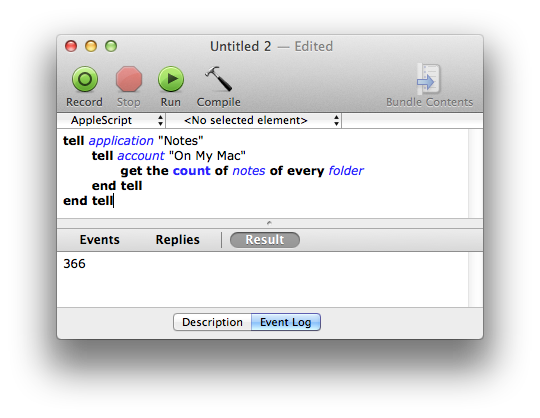
Example Scripts
The following script examples demonstrate how to access and manipulate the account class element. To open an example script in the AppleScript Editor application on your computer, click the script icon at the top left of an example script. (Requires OS X Mountain Lion)

This example demonstrates how to create a new note in a specific account. Note that the body of a note is actually stored and displayed as HTML data. So to preserve line breaks and formatting, the text from the clipboard is encased in HTML pre-formatting tags.